devtools
Dev tools
You can use Redux DevTools Extension for plain objects and arrays.
import { devtools } from 'valtio/utils'
const state = proxy({ count: 0, text: 'hello' })
const unsub = devtools(state, { name: 'state name', enabled: true })
Manipulating state with Redux DevTools
The screenshot below shows how to use Redux DevTools to manipulate state. First select the object from the instances drop down. Then type in a JSON object to dispatch. Then click "Dispatch". Notice how it changes the state.
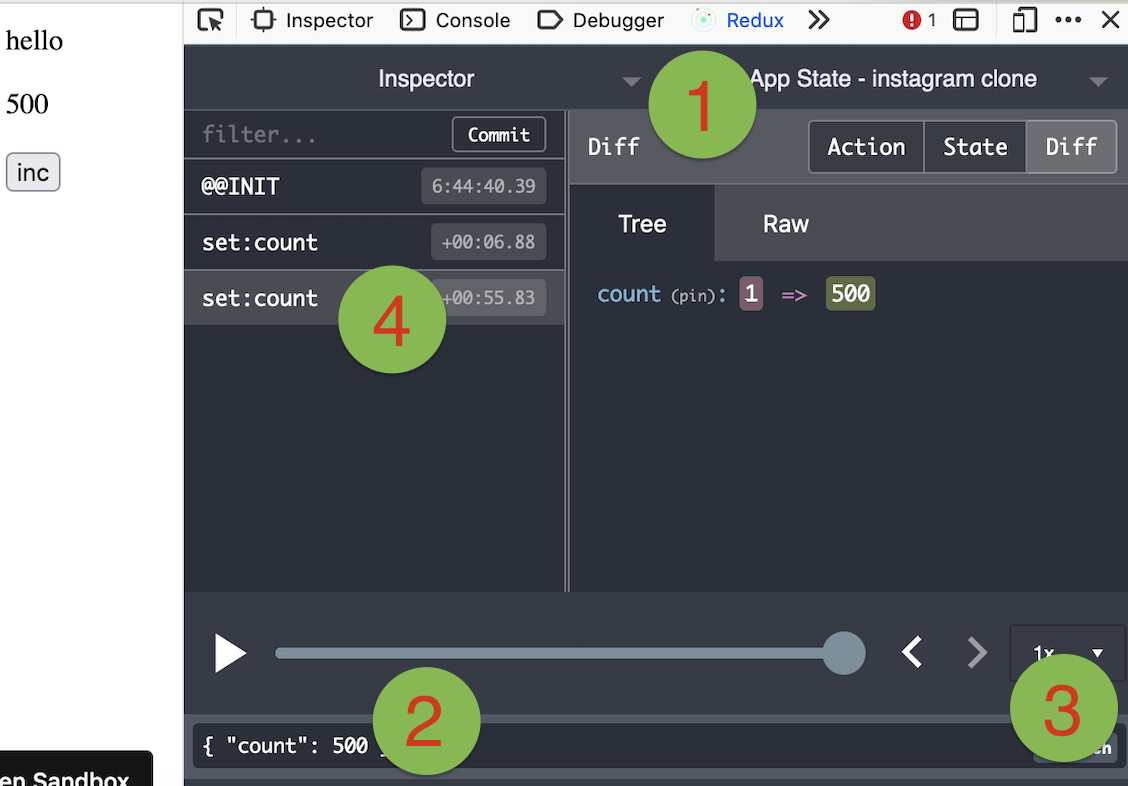
Use it with vanilla JS
Valtio is not tied to React, you can use it in vanillaJS.
```jsx
import { proxy, subscribe, snapshot } from 'valtio/vanilla'
const state = proxy({ count: 0, text: 'hello' })
subscribe(state, () => {
console.log('state is mutated')
const obj = snapshot(state) // A snapshot is an immutable object
})
Use it with TypeScript
It's recommended to install and import types from @redux-devtools/extension
to get types correctly.
import type {} from '@redux-devtools/extension'
import { devtools } from 'valtio/utils'